My son and his friend wanted to record as they play together on a bass and an electric guitar.
To record two instruments simultaneously in Garage Band you need special hardware to connect both to the computer. You can buy a USB Recording Interface for a few hundred dollars, or you can make one for $13 plus tax.
All you need is a computer, two electric instruments or microphones, and a simple audio cable.
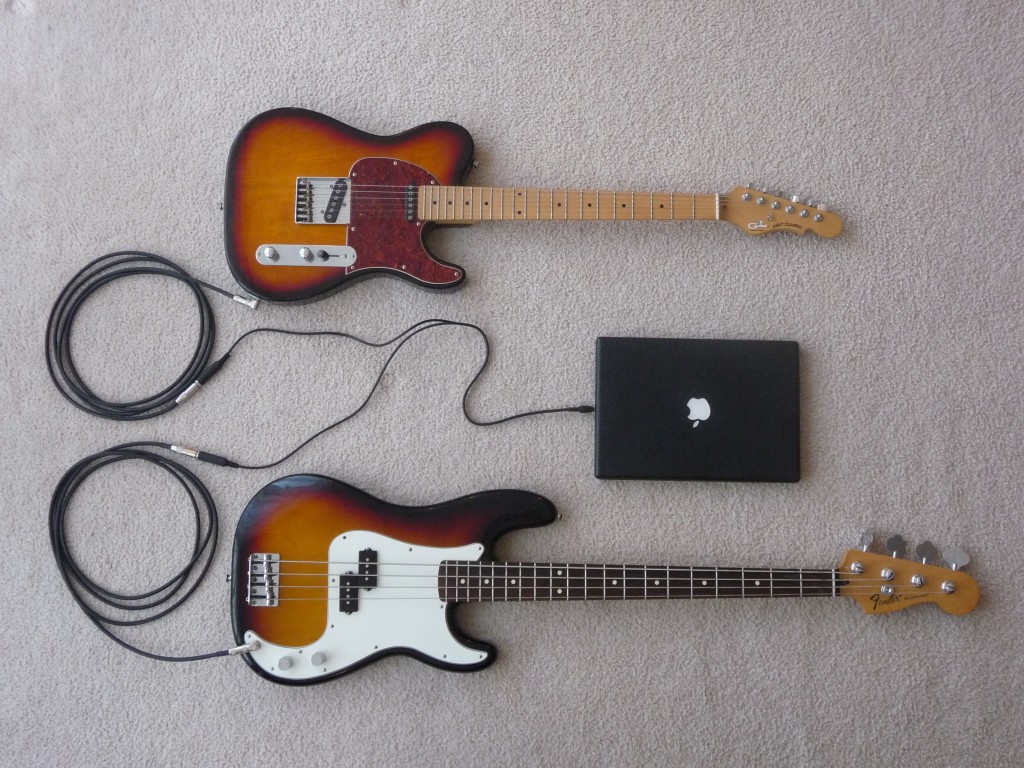
In this example we will connect a bass and an electric guitar to a Macintosh computer and record them simultaneously with Garage Band. Computers usually have only one microphone input connector, so you can only connect one instrument or microphone at a time. But these audio inputs have two channels (left and right), so the sound card can record two mono audio signals simultaneously. Basses, electric guitars and most microphones produce mono (one channel) signals, so we can connect one of them to the left input and the other to the right input of the computer. To connect two audio sources to the computer simultaneously you need a special audio cable with two mono 1/4″ phone female jacks on one end and a 1/8″ (3.5mm) stereo male plug on the other.
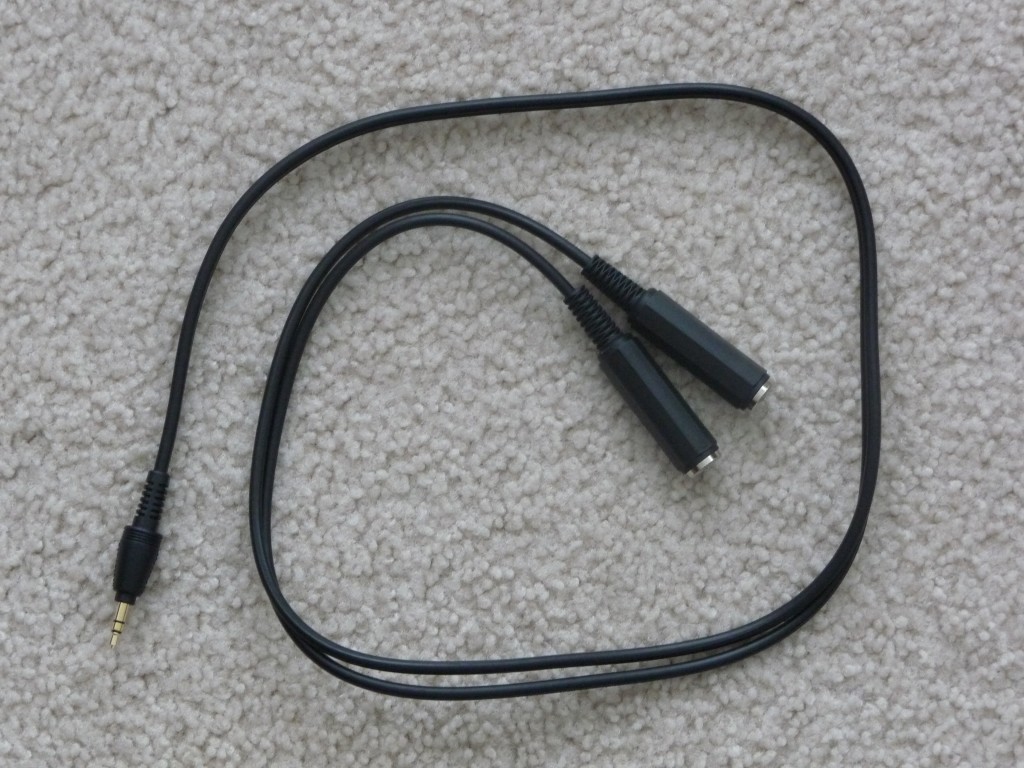
One 1/4″ phone female jack has to be connected to the left channel, the other to the right channel of the cable. If you cannot find a cable ready made in the stores you can make one yourself.
Make you own recording interface
You need some tools, that you can also buy at RadioShack.
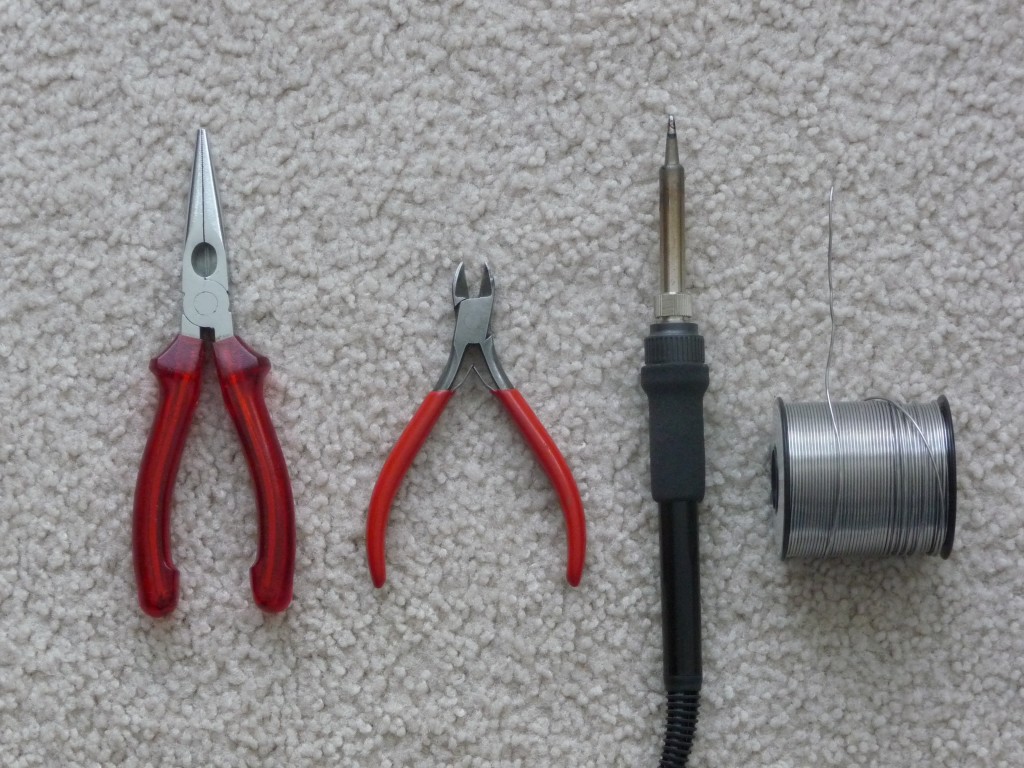
You need long-nose pliers, diagonal cutters, soldering iron and solder.
Buy
- one 3ft (91 cm) audio Y-cable with 1/8″ (3.5mm) stereo male plug on one end and dual RCA (Phono) male plugs on the other. (RadioShack catalog number 42-494)
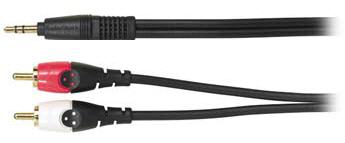
- one 2-pack 1/4″ mono female phone jacks (RadioShack catalog number 274-141)
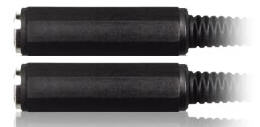
The 1/8″ stereo to dual RCA cable exposes the left and right channels of the audio input of the computer. The white plug is connected to the left channel, the red plug is connected to the right channel of the cable.
- Cut off the RCA male phono plugs and solder the 1/4″ phone jacks to the end of the cables.
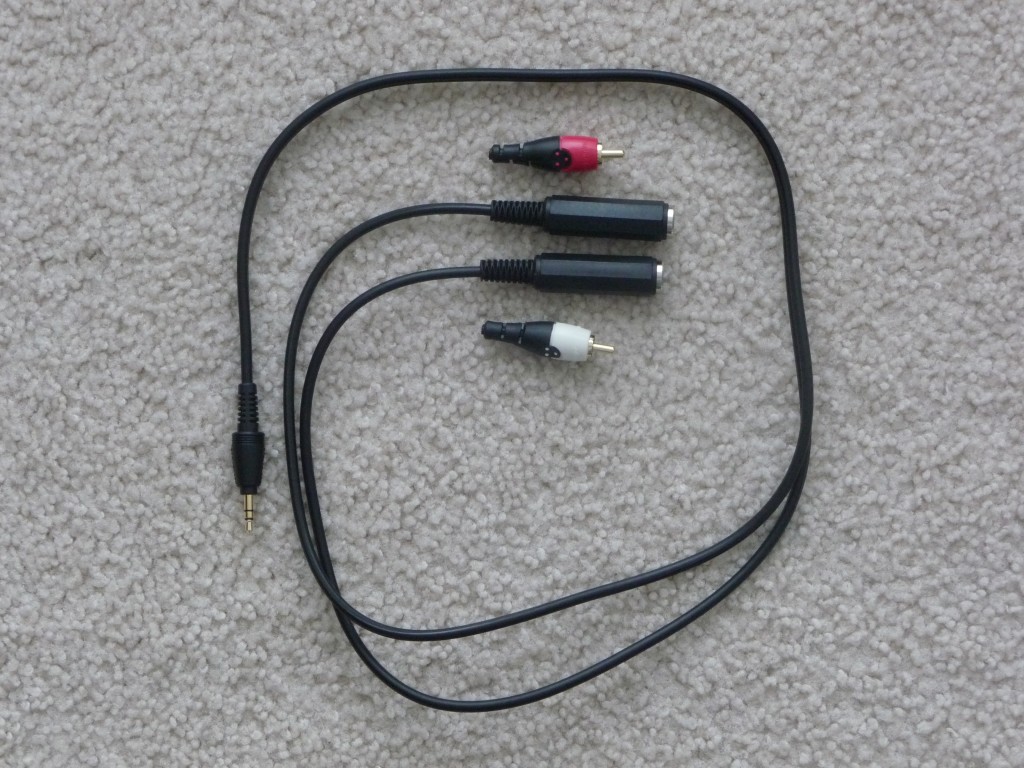
- To make the cable more durable you can use shrink tubes on the phone jack end.
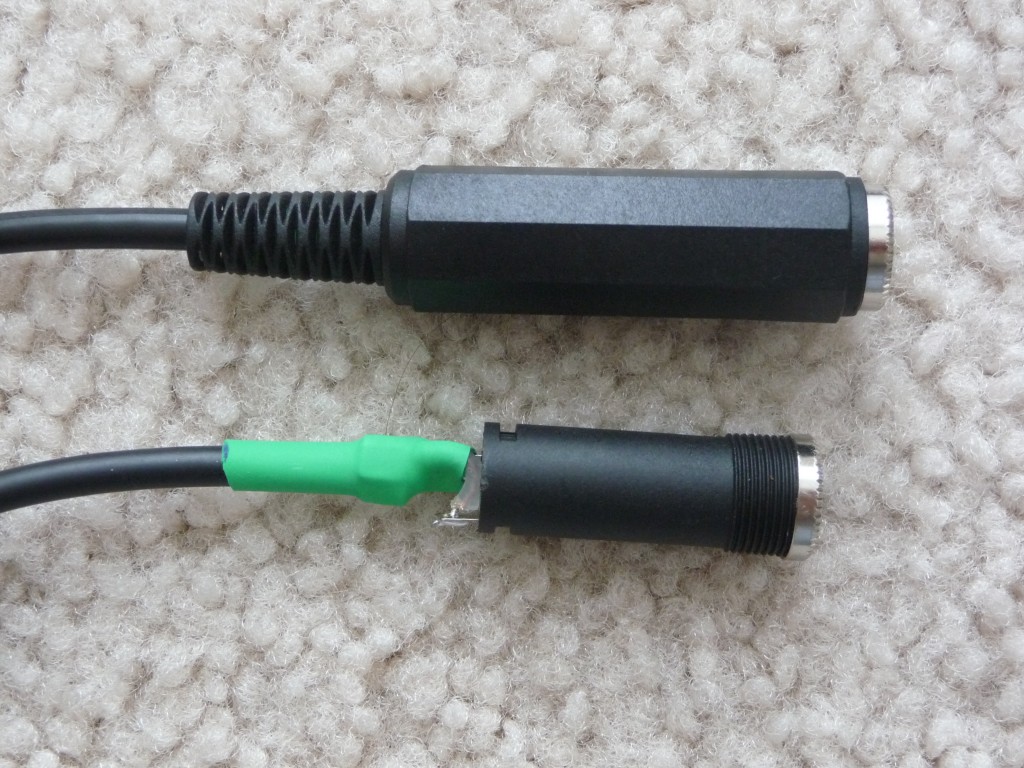
Connect the instruments to the computer
- Connect the bass to the left channel (where the white RCA plug was) and the electric guitar to the right channel (where the red RCA plug was) with standard guitar cables.
- Insert the 1/8″ stereo plug into the microphone input of the Macintosh
Record multiple instruments simultaneously
By default Garage Band can record only one track at a time, so we will enable multitrack recording in the Track menu of Garage Band below.
- Create a new Voice project
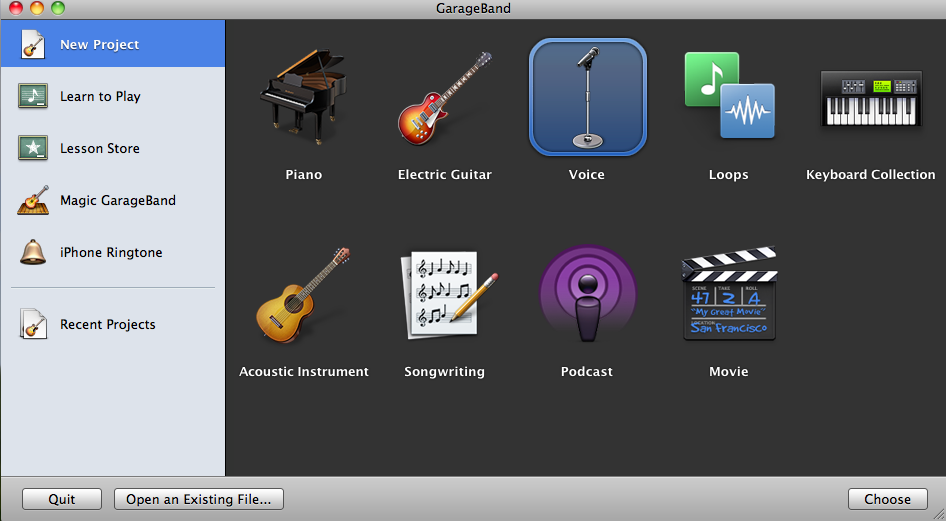
- Set the name, tempo, signature and key of the project and click Create
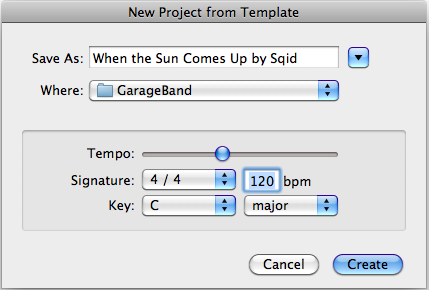
The new vocal project contains two tracks.
- On the left side select the first track and on the right side set the instrument to Bass
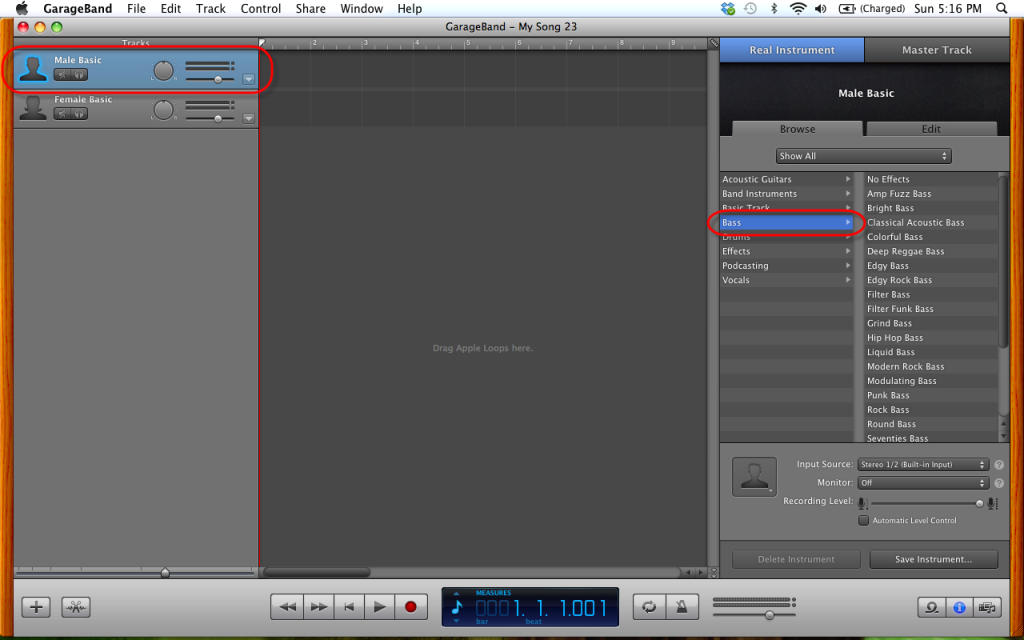
- On the right side set the desired bass effect
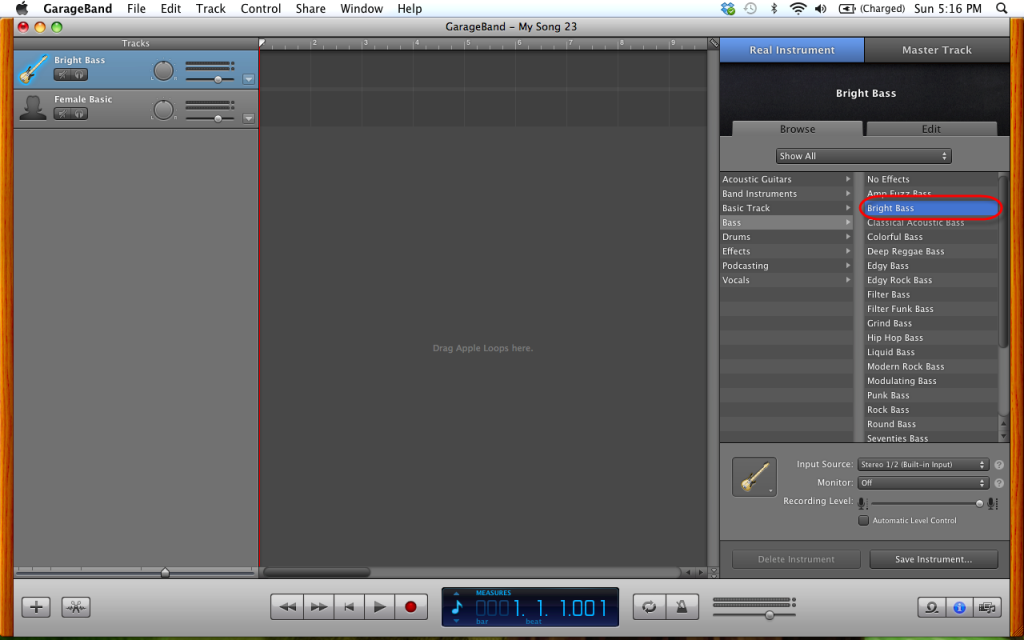
- Set the input to Mono 1 (Built-in Input). This is the left channel.
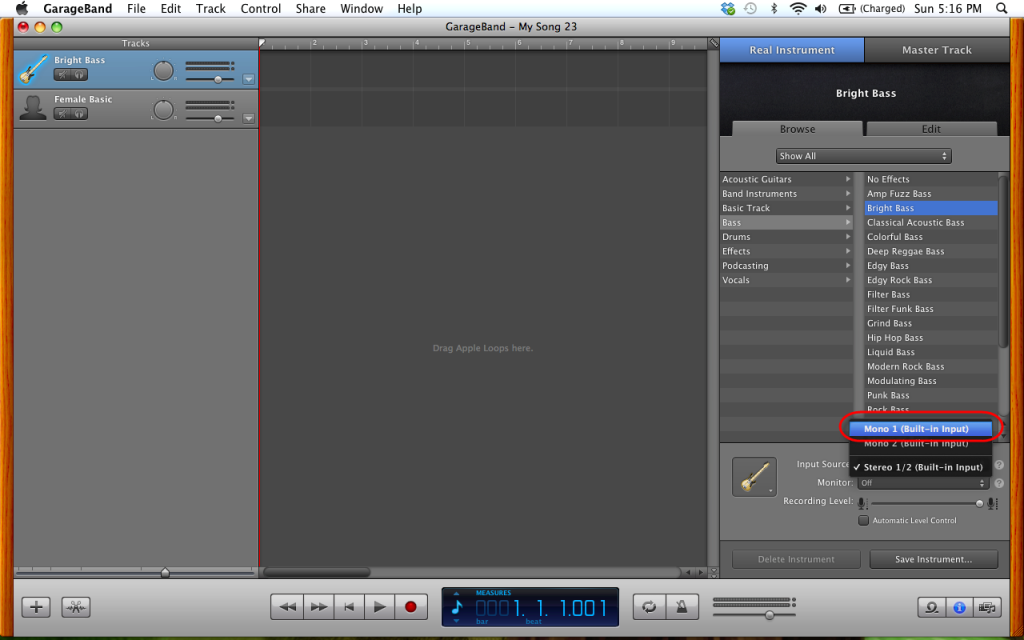
Turn on the track monitoring, so you can hear what you play.
- On the right side select On (no feedback protection)
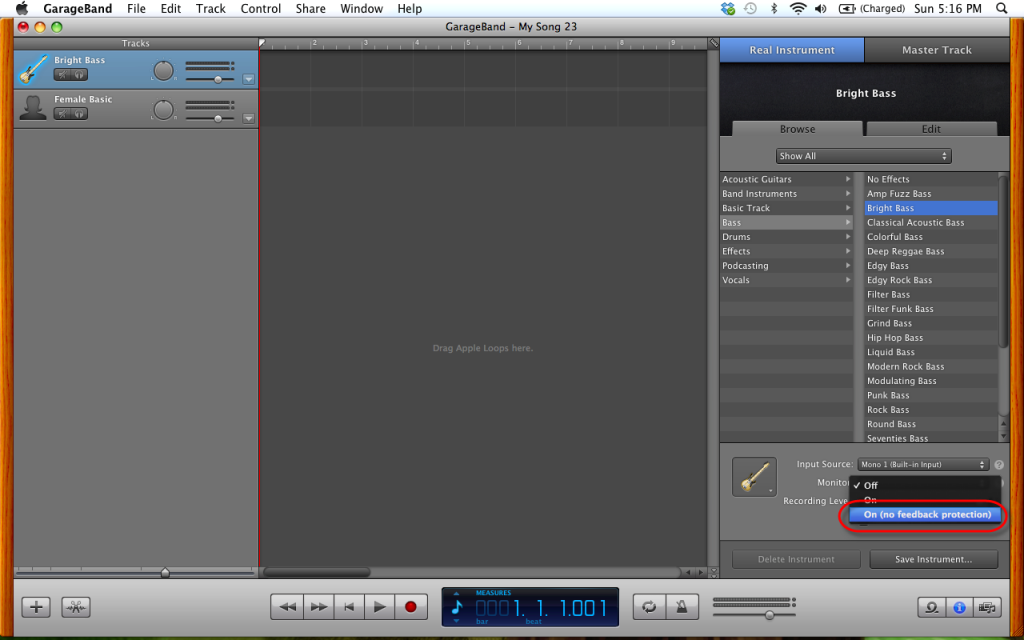
- To be able to simultaneously select multiple tracks for recording select Enable Multitrack Recording in the Track menu
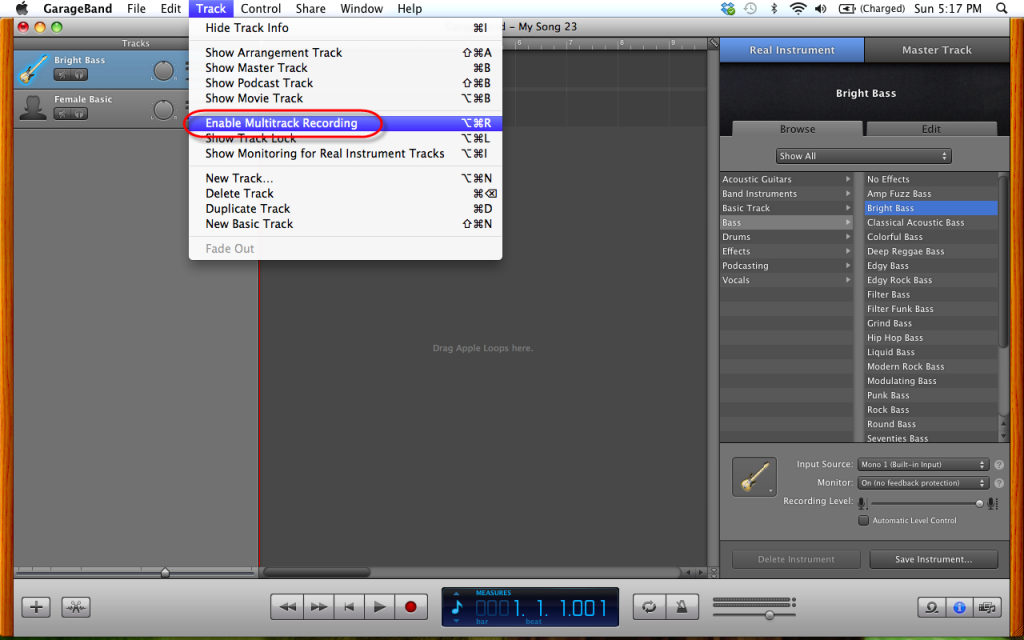
Set up the second track as Vocals and Guitar.
- In Garage Band every track needs its own input, so you have to set the input of the second track to Mono 2 (Built-in Input). This is the right channel.
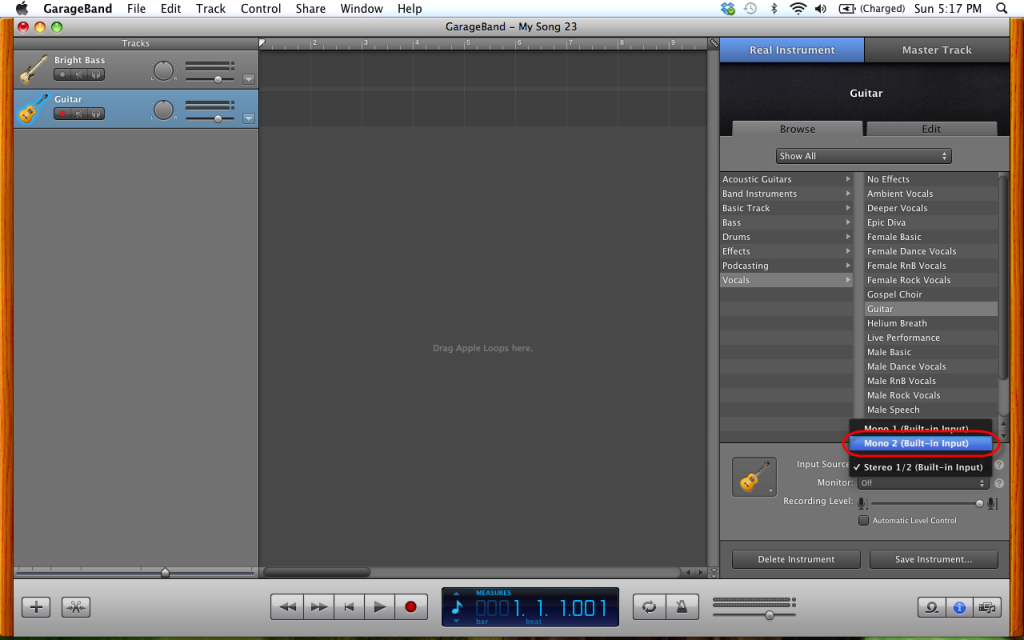
- To arm both tracks for recording on the left side select the red recording buttons for both tracks
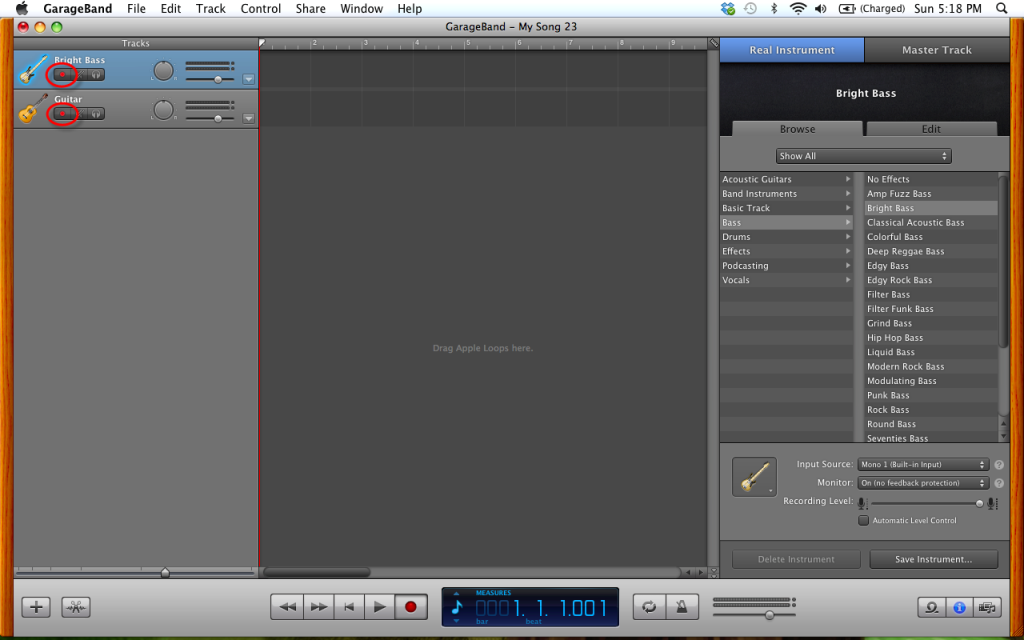